In Spring Boot there is an annotation @Async to assist developers for developing concurrent applications. But using this feature is quite tricky. In this blog we will see how to use this feature along with CompletableFuture. I assumed you know the drill about CompletableFuture, so I won’t repeat the concept here.
First of all you need to annotate your application class with @EnableAsync, this annotation tells the Spring to look for methods that are annotated with @Async and run them in a separate executor.
@SpringBootApplication @EnableAsync public class App { RestTemplate public static void main(String[] args) { SpringApplication.run(App.class, args); } }
If you take a look at Spring Boot example about @Async using CompletableFuture you’ll notice the way they’re using this feature is based on a REST request, in my opinion, I beleive, it’s kinda limited, it doesn’t give you a clue of how to use such feature in other situation. For an instance if you have a long running task what would you do about it?
// Source : https://spring.io/guides/gs/async-method/ package hello; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.boot.web.client.RestTemplateBuilder; import org.springframework.scheduling.annotation.Async; import org.springframework.stereotype.Service; import org.springframework.web.client.RestTemplate; import java.util.concurrent.CompletableFuture; @Service public class GitHubLookupService { private static final Logger logger = LoggerFactory.getLogger(GitHubLookupService.class); private final RestTemplate restTemplate; public GitHubLookupService(RestTemplateBuilder restTemplateBuilder) { this.restTemplate = restTemplateBuilder.build(); } @Async public CompletableFuture<User> findUser(String user) throws InterruptedException { logger.info("Looking up " + user); String url = String.format("https://api.github.com/users/%s", user); User results = restTemplate.getForObject(url, User.class); // Artificial delay of 1s for demonstration purposes Thread.sleep(1000L); return CompletableFuture.completedFuture(results); } }
In FindUser(String user), it uses a synthetic delay in the main thread also the main task of this method is fetching data from github using RestTemplate, this class is a “Synchronous client to perform HTTP requests”. How about using a long running task such as calling a network function, like ping a server from your REST endpoint? In that case you need to tailor the CompletableFuture. You can’t simply call following line and carry on.
return CompletableFuture.completedFuture(results);
How to Use CompletableFuture
For using @Async in your code, your method has to return Future or CompletableFuture for more information you can refer to its document. Take a look at following example :
@Async public CompletableFuture<Boolean> isServerAlive(String ip) { CompletableFuture<Boolean> future = new CompletableFuture<Boolean>(){ @Override public Boolean get() throws InterruptedException, ExecutionException { InetAddress address = null; try { address = InetAddress.getByName(ip); return address.isReachable(1000); } catch (UnknownHostException e) { e.printStackTrace(); return false; } catch (IOException e) { e.printStackTrace(); return false; } } }; return future; }
In this example I override the get() method and return the CompletableFuture without any thread executor, in fact with this method we ask Spring to execute the @Async method in a different thread, but we don’t provide any thread executor, only body of a background-worker will suffice.
download source code from github
P.S : In this example I decided to use a network function inside Spring Boot just for the sake of argument. But it’s better to not to use network functions directly in a REST endpoint. Specially when you expect to get an immediate result out of it. The reason: network functions are blocking which means, if you call this REST endpoint. You’ll have to wait to get the result from the endpoint. It’s highly advised to use other methods such as queue or push method(e.g. websocket) for calling blocking functions.
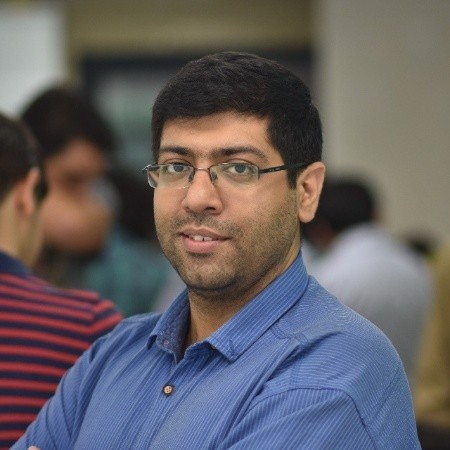
My chief interest is software engineering, mainly dealing with Linux and Java projects, but also interested in teaching what I’ve learned, have published a couple of CBTs about programming in Persian (java/Android) and here I’ll share my thoughts and experiences in English as well.
Leave a Reply