If you are working in an enterprise infrastructures, chances are that you are using a centralized authentication system, most likely Active Directory or openLDAP. In this blog I’ll explore how to create a REST API using spring boot to authenticate against openLDAP and create a JWT token in return.
Before getting our hand dirty, we need to review the architecture of spring security and the way we want to utilise it, in a REST API endpoint. According to openLDAP, I’ve explained it’s concept briefly before, you can read more about it here. Also I’ll assume that you know how Spring Boot and JWT works.
Spring Security
In this example I will extend the WebSecurityConfigurerAdapter. This class will assist me to intercept security chain of spring security and insert openLDAP authentication adapter in between.
In fact this abstract class provides convenient methods for configuring spring security configuration using HTTPSecurity object.
First of all I injected three different beans as follows :
private OpenLdapAuthenticationProvider openLdapAuthenticationProvider; private JwtAuthenticationEntryPoint jwtAuthenticationEntryPoint; private JwtRequestFilter jwtRequestFilter;
Then override the configure(AuthenticationManagerBuilder auth) :
@Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { auth.authenticationProvider(openLdapAuthenticationProvider); }
This will let us to override default behaviour of spring security authentication. In addition we need to override the configure(HttpSecurity httpSecurity):
@Override protected void configure(HttpSecurity httpSecurity) throws Exception { // We don't need CSRF for this example httpSecurity .csrf().disable() .headers() .frameOptions() .deny() .and() // dont authenticate this particular request .authorizeRequests().antMatchers("/api/login").permitAll(). // all other requests need to be authenticated antMatchers("/api/**").authenticated().and(). // make sure we use stateless session; session won't be used to // store user's state. exceptionHandling().authenticationEntryPoint(jwtAuthenticationEntryPoint).and().sessionManagement() .sessionCreationPolicy(SessionCreationPolicy.STATELESS); // Add a filter to validate the tokens with every request httpSecurity.addFilterBefore(jwtRequestFilter, UsernamePasswordAuthenticationFilter.class); }
Also For the sake of manually authenticating a user in /api/login we will expose the authenticationManagerBean() :
@Bean @Override public AuthenticationManager authenticationManagerBean() throws Exception { return super.authenticationManagerBean(); }
After configuring WebSecurityConfig, I’ll provide my customer authentication adapter. This adapter will utilise spring’s LdapTemplate and let us to establish a connection to a LDAP server.
@Component public class OpenLdapAuthenticationProvider implements AuthenticationProvider { @Autowired private LdapContextSource contextSource; private LdapTemplate ldapTemplate; @PostConstruct private void initContext() { contextSource.setUrl("ldap://1.1.1.1:389/ou=users,dc=www,dc=devcrutch,dc=com"); // I use anonymous binding so, no need to provide bind user/pass contextSource.setAnonymousReadOnly(true); contextSource.setUserDn("ou=users,"); contextSource.afterPropertiesSet(); ldapTemplate = new LdapTemplate(contextSource); } @Override public Authentication authenticate(Authentication authentication) throws AuthenticationException { Filter filter = new EqualsFilter("uid", authentication.getName()); Boolean authenticate = ldapTemplate.authenticate(LdapUtils.emptyLdapName(), filter.encode(), authentication.getCredentials().toString()); if (authenticate) { List<GrantedAuthority> grantedAuthorities = new ArrayList<>(); grantedAuthorities.add(new SimpleGrantedAuthority("ROLE_USER")); UserDetails userDetails = new User(authentication.getName() ,authentication.getCredentials().toString() ,grantedAuthorities); Authentication auth = new UsernamePasswordAuthenticationToken(userDetails, authentication.getCredentials().toString() , grantedAuthorities); return auth; } else { return null; } } @Override public boolean supports(Class<?> authentication) { return authentication.equals(UsernamePasswordAuthenticationToken.class); } }
Another part that we have to take into consideration is, implementing user login controller. Since we haven’t provided any filter for controlling username and password we ought to implementing it manually as follows :
@RestController @RequestMapping("/api/login") public class LoginController { @Autowired private AuthenticationManager authenticationManager; @Autowired private JwtTokenUtil jwtTokenUtil; @Autowired private UserService userService; @PostMapping public ResponseEntity<?> createAuthenticationToken(@RequestBody JwtRequest authenticationRequest) throws Exception { authenticate(authenticationRequest.getUsername(), authenticationRequest.getPassword()); final User userDetails = userService.loadUserByUsername(authenticationRequest.getUsername()); final String token = jwtTokenUtil.generateToken(userDetails); return ResponseEntity.ok(new JwtResponse(token)); } private void authenticate(String username, String password) throws Exception { try { authenticationManager.authenticate(new UsernamePasswordAuthenticationToken(username, password)); } catch (DisabledException e) { throw new Exception("USER_DISABLED", e); } catch (BadCredentialsException e) { throw new Exception("INVALID_CREDENTIALS", e); } } }
These are the pillars of having a REST API + JWT + LDAP back-end using spring boot.
Now we can test the API using a REST client.
After getting the JWT token we can call authorized endpoints
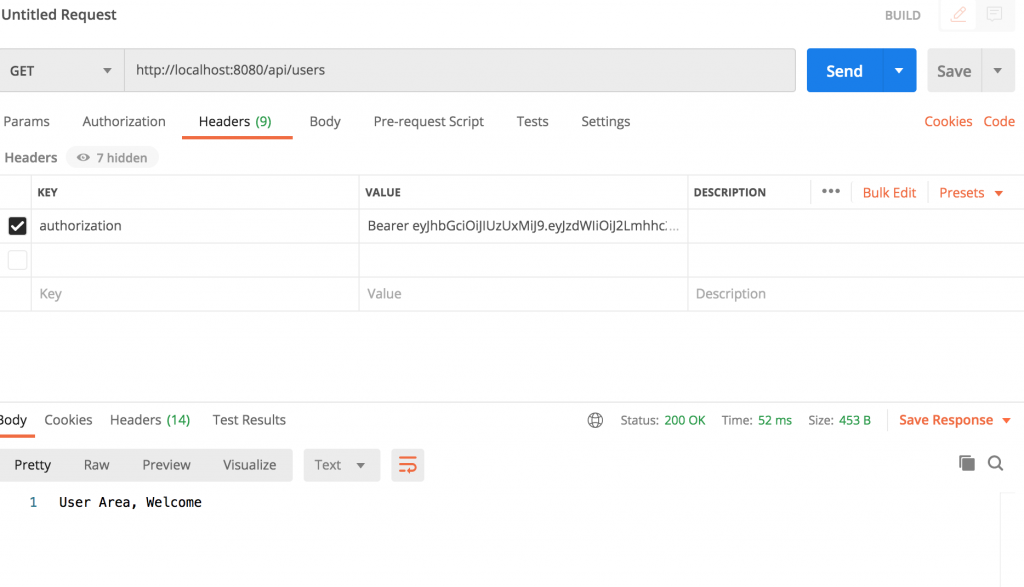
You can find a working source code on my github.
For the next part I’ll make this code more concise.
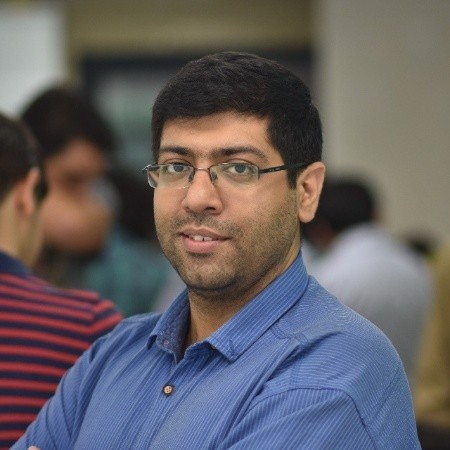
My chief interest is software engineering, mainly dealing with Linux and Java projects, but also interested in teaching what I’ve learned, have published a couple of CBTs about programming in Persian (java/Android) and here I’ll share my thoughts and experiences in English as well.
Leave a Reply